接下來, 改動一下代碼, 將有更明確的理解. 這裡有兩個Activity, 第一個AndroidRandom選擇constructor和seek, 第二個RunRandom從而建構一個隨機數字產生器, 如果繼續點擊便會繼續產生下一個隨機數字.

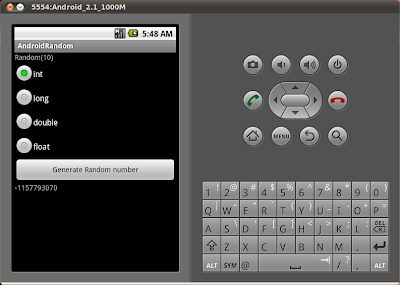
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello"
/>
<RadioGroup
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:checkedButton="@+id/milliseconds"
android:id="@+id/contructor" >
<RadioButton
android:text="Random()"
android:id="@+id/milliseconds" />
<RadioButton
android:text="Random(long seed)"
android:id="@+id/seed" />
</RadioGroup>
<EditText
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:inputType="number"
android:id="@+id/seeknumber"
/>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Construct a Random Generate"
android:id="@+id/construct"
/>
</LinearLayout>
AndroidRandom.java
package com.AndroidRandom;
import java.util.Random;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.TextView;
public class AndroidRandom extends Activity {
RadioButton rbRandomMilliseconds, rbRandomSeek;
EditText etSeekNumber;
Button buttonConstruct;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
rbRandomMilliseconds = (RadioButton)findViewById(R.id.milliseconds);
rbRandomSeek = (RadioButton)findViewById(R.id.seed);
etSeekNumber = (EditText)findViewById(R.id.seeknumber);
buttonConstruct = (Button)findViewById(R.id.construct);
buttonConstruct.setOnClickListener(buttonConstructOnClickListener);
}
private Button.OnClickListener buttonConstructOnClickListener
= new Button.OnClickListener(){
@Override
public void onClick(View v) {
String strTypeOfConstructor, strInitSeek;
// TODO Auto-generated method stub
if (rbRandomMilliseconds.isChecked()){
strTypeOfConstructor = "RandomMilliseconds";
}
else{
strTypeOfConstructor = "RandomSeek";
}
strInitSeek = etSeekNumber.getText().toString();
Intent intent = new Intent(AndroidRandom.this,RunRandom.class);
Bundle bundle = new Bundle();
bundle.putString("keyTypeOfConstructor", strTypeOfConstructor);
bundle.putString("keyInitSeek", strInitSeek);
intent.putExtras(bundle);
startActivity(intent);
}
};
}
runrandom.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/typeofconstructor"
/>
<RadioGroup
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:checkedButton="@+id/typeint"
android:id="@+id/type" >
<RadioButton
android:text="int"
android:id="@+id/typeint" />
<RadioButton
android:text="long"
android:id="@+id/typelong" />
<RadioButton
android:text="double"
android:id="@+id/typedouble" />
<RadioButton
android:text="float"
android:id="@+id/typefloat" />
</RadioGroup>
<Button
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Generate Random number"
android:id="@+id/generate"
/>
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/generatednumber"
/>
</LinearLayout>
RunRandom.java
package com.AndroidRandom;
import java.util.Random;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RadioButton;
import android.widget.TextView;
public class RunRandom extends Activity {
TextView tvTypeofconstructor;
RadioButton rbInt, rbLong, rbDouble, rbFloat;
Button buttonGenerate;
TextView textGeneratedNumber;
Long initSeek;
Random myRandom;
@Override
protected void onCreate(Bundle savedInstanceState) {
// TODO Auto-generated method stub
super.onCreate(savedInstanceState);
setContentView(R.layout.runrandom);
tvTypeofconstructor = (TextView)findViewById(R.id.typeofconstructor);
rbInt = (RadioButton)findViewById(R.id.typeint);
rbLong = (RadioButton)findViewById(R.id.typelong);
rbDouble = (RadioButton)findViewById(R.id.typedouble);
rbFloat = (RadioButton)findViewById(R.id.typefloat);
buttonGenerate = (Button)findViewById(R.id.generate);
textGeneratedNumber = (TextView)findViewById(R.id.generatednumber);
buttonGenerate.setOnClickListener(buttonGenerateOnClickListener);
Bundle bundle = this.getIntent().getExtras();
String typeOfConstructor = bundle.getString("keyTypeOfConstructor");
String strInitSeek = bundle.getString("keyInitSeek");
if (typeOfConstructor.equals("RandomMilliseconds")){
tvTypeofconstructor.setText("Random()");
myRandom = new Random();
}
else{
Long tSeek;
if (strInitSeek.equals(""))
{
tvTypeofconstructor.setText("Random(0)");
tSeek = 0L;
}
else
{
tvTypeofconstructor.setText("Random(" + strInitSeek + ")");
tSeek = Long.decode(strInitSeek);
}
myRandom = new Random(tSeek);
}
}
private Button.OnClickListener buttonGenerateOnClickListener
= new Button.OnClickListener(){
@Override
public void onClick(View v) {
if (rbInt.isChecked()){
textGeneratedNumber.setText(String.valueOf(myRandom.nextInt()));
}
else if (rbLong.isChecked()){
textGeneratedNumber.setText(String.valueOf(myRandom.nextLong()));
}
else if (rbDouble.isChecked()){
textGeneratedNumber.setText(String.valueOf(myRandom.nextDouble()));
}
else{
textGeneratedNumber.setText(String.valueOf(myRandom.nextFloat()));
}
}
};
}
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.AndroidRandom"
android:versionCode="1"
android:versionName="1.0">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".AndroidRandom"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".RunRandom"></activity>
</application>
<uses-sdk android:minSdkVersion="4" />
</manifest>
沒有留言:
發佈留言