package com.example.androidmemoryinfo; import android.os.Bundle; import android.app.Activity; import android.app.ActivityManager; import android.app.ActivityManager.MemoryInfo; import android.widget.TextView; public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); //setContentView(R.layout.activity_main); TextView tv = new TextView(this); setContentView(tv); ActivityManager activityManager = (ActivityManager)getSystemService(ACTIVITY_SERVICE); MemoryInfo memoryInfo = new MemoryInfo(); activityManager.getMemoryInfo(memoryInfo); String info = "available memory : " + String.valueOf(memoryInfo.availMem) + "\n" + "currently be in a low memory : " + String.valueOf(memoryInfo.lowMemory) + "\n" + "threshold low memory : " + String.valueOf(memoryInfo.threshold) + "\n" + "total memory accessible by the kernel : " + String.valueOf(memoryInfo.totalMem); tv.setText(info); } }
2012年12月28日星期五
讀取內存信息, MemoryInfo
我們可以通過 android.app.ActivityManager.MemoryInfo 讀取內存信息.
2012年12月4日星期二
谷歌推出新的遊戲服務, Google Play services v2.0, 包括新的Google Maps Android API.
谷歌遊戲服務 (Google Play services) 是整合谷歌產品的新平台, 為應用程序提供更大的靈活性. 谷歌推出新的遊戲服務, Google Play services v2.0, 其中還包括新的Google Maps Android API.
新版本的API,讓開發者在應用程序使用很多Android谷歌地圖 (Google Maps for Android) 的最新功能.
詳細內容: New Google Maps Android API now part of Google Play services
新版本的API,讓開發者在應用程序使用很多Android谷歌地圖 (Google Maps for Android) 的最新功能.
詳細內容: New Google Maps Android API now part of Google Play services
2012年11月14日星期三
2012年10月19日星期五
谷歌數據中心(Google Data Center) 內望
你想看看谷歌數據中心(Google Data Center)內的情況嗎? 現在你通過谷歌街景(Street View)參觀谷歌的數據中心.
http://www.google.com/about/datacenters/streetview
http://www.google.com/about/datacenters/streetview
2012年10月5日星期五
如何在 TextView 顯示浮點數(float), 並且指定小數點後位數目
要顯示浮點數(float), 並且指定小數點後位數目, 可以使用 String.format() 方法.
實例:
在 TextView 顯示浮點數(myfloatNumber), 並且指定顯示小數點後二位.
textview.setText(String.format("%.02f", myfloatNumber));
實例:
在 TextView 顯示浮點數(myfloatNumber), 並且指定顯示小數點後二位.
textview.setText(String.format("%.02f", myfloatNumber));
2012年9月11日星期二
2012年9月9日星期日
2012年8月4日星期六
2012年7月30日星期一
2012年7月11日星期三
2012年6月30日星期六
Google I/O 2012 大會視頻 - What's New in Android Developers' Tools
本視頻介紹最新的 Android SDK Revision 20 的新功能.
2012年6月28日星期四
谷歌官方發佈 Android 4.1, Jelly Bean (果凍豆)
谷歌已在開發者博客(http://android-developers.blogspot.com/2012/06/introducing-android-41-jelly-bean.html)官方發佈 Android 4.1, Jelly Bean (果凍豆).
相關文件:
- Android 4.1 for Developers
- Android 4.1 APIs
相關文件:
- Android 4.1 for Developers
- Android 4.1 APIs
2012年6月7日星期四
使用通知生成器 (Notification.Builder) 創建通知 (Notification)
Android 3.0, API Level 11, 提供新的 android.app.Notification.Builder 類. 用於更容易創建通知(Notification).
實例:
因為這個例子創建的通知會產生震動, 因此需要修改 AndroidManifest.xml, 添加 "android.permission.VIBRATE" 許可.
實例:
package com.Android3NotificationBuilder; import android.app.Activity; import android.app.Notification; import android.app.NotificationManager; import android.app.PendingIntent; import android.content.Context; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; public class Android3NotificationBuilderActivity extends Activity { Button buttonCreateNotification; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); buttonCreateNotification = (Button)findViewById(R.id.createnotification); buttonCreateNotification.setOnClickListener( new Button.OnClickListener(){ @Override public void onClick(View arg0) { createNotification(getBaseContext()); }} ); } private void createNotification(Context context){ NotificationManager notificationManager = (NotificationManager)context.getSystemService( Context.NOTIFICATION_SERVICE); Notification.Builder builder = new Notification.Builder(context); Intent intent = new Intent(context, Android3NotificationBuilderActivity.class); PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, 0); long[] vibratepattern = {100, 400, 500, 400}; builder .setSmallIcon(R.drawable.ic_launcher) .setContentTitle("標題") .setContentText("文字") .setContentInfo("信息") .setTicker("票") .setLights(0xFFFFFFFF, 1000, 1000) .setVibrate(vibratepattern) .setContentIntent(pendingIntent) .setAutoCancel(false); Notification notification = builder.getNotification(); notificationManager.notify(R.drawable.ic_launcher, notification); } }
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> <Button android:id="@+id/createnotification" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="創建通知(Notification)" /> </LinearLayout>
因為這個例子創建的通知會產生震動, 因此需要修改 AndroidManifest.xml, 添加 "android.permission.VIBRATE" 許可.
2012年6月5日星期二
振動器 (Vibrator)
我們可以使用 android.os.Vibrator 類控制 Android 設備上的振動器.
實例:
使用 android.os.Vibrator (振動器), 需要修改 AndroidManifest.xml 添加 "android.permission.VIBRATE" 權限.
- boolean hasVibrator(): 檢查硬件是否有震動器. (API Level 11)
- void vibrate(long milliseconds): 開啟振動器.
- void vibrate(long[] pattern, int repeat): 按模式振動.
- void cancel(): 取消振動.
實例:
package com.AndroidVibrator; import android.app.Activity; import android.os.Bundle; import android.os.Vibrator; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; public class AndroidVibratorActivity extends Activity { long[] vibratepattern = {100, 400, 500}; Vibrator vibrator; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); vibrator = (Vibrator)getSystemService( AndroidVibratorActivity.VIBRATOR_SERVICE); Button startVibrate = (Button)findViewById(R.id.startvibrate); startVibrate.setOnClickListener(new OnClickListener(){ @Override public void onClick(View arg0) { vibrator.vibrate(vibratepattern, 0); }}); Button stopVibrate = (Button)findViewById(R.id.stopvibrate); stopVibrate.setOnClickListener(new OnClickListener(){ @Override public void onClick(View v) { vibrator.cancel(); }}); } }
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> <Button android:id="@+id/startvibrate" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Start Vibrate" /> <Button android:id="@+id/stopvibrate" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Stop Vibrate" /> </LinearLayout>
使用 android.os.Vibrator (振動器), 需要修改 AndroidManifest.xml 添加 "android.permission.VIBRATE" 權限.
2012年5月30日星期三
2012年5月24日星期四
在百度地图(Baidu Map)顯示我的位置(MyLocation)和指南針(Compass)
只要在百度地图(Baidu Map)的地圖視圖(MapView)添加我的位置覆蓋(MyLocationOverlay), 便可以很容易顯示我的位置和指南針.
示例代碼:
MKLocationManager mLocationManager = mBMapMan.getLocationManager();
mLocationManager.enableProvider(MKLocationManager.MK_NETWORK_PROVIDER);
mLocationManager.enableProvider(MKLocationManager.MK_GPS_PROVIDER);
MyLocationOverlay myLocationOverlay = new MyLocationOverlay(this, mMapView);
myLocationOverlay.enableMyLocation();
myLocationOverlay.enableCompass();
mMapView.getOverlays().add(myLocationOverlay);
修改前文"編寫簡單的百度地图(Baidu Map)應用程序"的 BaiduMapActivity.java
示例代碼:
MKLocationManager mLocationManager = mBMapMan.getLocationManager();
mLocationManager.enableProvider(MKLocationManager.MK_NETWORK_PROVIDER);
mLocationManager.enableProvider(MKLocationManager.MK_GPS_PROVIDER);
MyLocationOverlay myLocationOverlay = new MyLocationOverlay(this, mMapView);
myLocationOverlay.enableMyLocation();
myLocationOverlay.enableCompass();
mMapView.getOverlays().add(myLocationOverlay);
修改前文"編寫簡單的百度地图(Baidu Map)應用程序"的 BaiduMapActivity.java
package com.BaiduMap; import com.baidu.mapapi.BMapManager; import com.baidu.mapapi.GeoPoint; import com.baidu.mapapi.MKLocationManager; import com.baidu.mapapi.MapActivity; import com.baidu.mapapi.MapController; import com.baidu.mapapi.MapView; import com.baidu.mapapi.MyLocationOverlay; import android.os.Bundle; public class BaiduMapActivity extends MapActivity { //使用你自己的密鑰 //參考準備工作 http://goo.gl/j6HkK final static String MY_KEY = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"; BMapManager mBMapMan; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); mBMapMan = new BMapManager(getApplication()); mBMapMan.init(MY_KEY, null); super.initMapActivity(mBMapMan); MapView mMapView = (MapView) findViewById(R.id.bmapsView); mMapView.setBuiltInZoomControls(true); MapController mMapController = mMapView.getController(); GeoPoint point = new GeoPoint((int) (39.915 * 1E6), (int) (116.404 * 1E6)); mMapController.setCenter(point); mMapController.setZoom(12); //Add MyLocationOverlay MKLocationManager mLocationManager = mBMapMan.getLocationManager(); mLocationManager.enableProvider(MKLocationManager.MK_NETWORK_PROVIDER); mLocationManager.enableProvider(MKLocationManager.MK_GPS_PROVIDER); MyLocationOverlay myLocationOverlay = new MyLocationOverlay(this, mMapView); myLocationOverlay.enableMyLocation(); myLocationOverlay.enableCompass(); mMapView.getOverlays().add(myLocationOverlay); } @Override protected boolean isRouteDisplayed() { // TODO Auto-generated method stub return false; } @Override protected void onDestroy() { if (mBMapMan != null) { mBMapMan.destroy(); mBMapMan = null; } super.onDestroy(); } @Override protected void onPause() { if (mBMapMan != null) { mBMapMan.stop(); } super.onPause(); } @Override protected void onResume() { if (mBMapMan != null) { mBMapMan.start(); } super.onResume(); } }
2012年5月23日星期三
編寫簡單的百度地图(Baidu Map)應用程序
完成前文的準備工作, 現在我們可以動手編寫百度地图應用程序.
- 修改 main.xml, 加入 com.baidu.mapapi.MapView, 這是百度地图的視圖.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> <com.baidu.mapapi.MapView android:id="@+id/bmapsView" android:layout_width="fill_parent" android:layout_height="fill_parent" android:clickable="true" /> </LinearLayout>
- 修改活動的主要 Java 代碼, 注意它是擴展 MapActivity, 不是 Activity. MY_KEY字串須使用你自己的密鑰, 參考前文"準備工作".
package com.BaiduMap; import com.baidu.mapapi.BMapManager; import com.baidu.mapapi.GeoPoint; import com.baidu.mapapi.MapActivity; import com.baidu.mapapi.MapController; import com.baidu.mapapi.MapView; import android.os.Bundle; public class BaiduMapActivity extends MapActivity { //使用你自己的密鑰 //參考準備工作 http://goo.gl/j6HkK final static String MY_KEY = "xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx"; BMapManager mBMapMan; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); mBMapMan = new BMapManager(getApplication()); mBMapMan.init(MY_KEY, null); super.initMapActivity(mBMapMan); MapView mMapView = (MapView) findViewById(R.id.bmapsView); mMapView.setBuiltInZoomControls(true); MapController mMapController = mMapView.getController(); GeoPoint point = new GeoPoint((int) (39.915 * 1E6), (int) (116.404 * 1E6)); mMapController.setCenter(point); mMapController.setZoom(12); } @Override protected boolean isRouteDisplayed() { // TODO Auto-generated method stub return false; } @Override protected void onDestroy() { if (mBMapMan != null) { mBMapMan.destroy(); mBMapMan = null; } super.onDestroy(); } @Override protected void onPause() { if (mBMapMan != null) { mBMapMan.stop(); } super.onPause(); } @Override protected void onResume() { if (mBMapMan != null) { mBMapMan.start(); } super.onResume(); } }
相關文章:
- 在百度地图(Baidu Map)顯示我的位置(MyLocation)和指南針(Compass)
2012年5月22日星期二
在Android應用程序使用百度地图(Baidu Map) - 準備工作
類似谷歌地圖(Google Maps), 百度地图移动版API(Android)是一套基于Android 1.5及以上版本设备的应用程序接口,通过该接口,您可以轻松访问百度服务和数据,构建功能丰富、交互性强的地图应用程序。百度地图移动版API不仅包含构建地图的基本接口,还提供了诸如本地搜索、路线规划等数据服务,你可以根据自己的需要进行选择。
在動手編寫百度地图應用程序以前, 我們必須準備一些工作:
- 申请Key: 在使用API之前需要获取百度地图移动版API Key,该Key与你的百度账户相关联,您必须先有百度帐户,才能获得API KEY。并且,该KEY与您引用API的程序名称有关,具体流程请参照获取密钥。请妥善保存Key,地图初始化时需要用到Key。
- 把API开发包添加到Android工程的libs中: 瀏覽Android平台/相关下载網頁, 點擊API开发包下载Android工程中引用的jar和so文件。首先在工程里新建libs文件夹,并API开发包里的baidumapapi.jar拷贝到libs根目录下,将libBMapApiEngine.so拷贝到libs\armeabi目录下。
- 添加API开发包入工程的構建路徑:
右擊工程, 選擇 Properties.
選擇 Java Build Path, 按Add JARS...按鈕.
打開工程下面的libs文件夾,選擇baidumapapi.jar, 按OK按鈕.
再按OK按鈕完成.
- 修改AndroidManifest.xml添加使用权限Android版本支持:
<supports-screens android:largeScreens="true" android:normalScreens="true" android:smallScreens="true" android:resizeable="true" android:anyDensity="true"/> <uses-sdk android:minSdkVersion="3" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"></uses-permission> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"></uses-permission> <uses-permission android:name="android.permission.INTERNET"></uses-permission> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"></uses-permission> <uses-permission android:name="android.permission.ACCESS_WIFI_STATE"></uses-permission> <uses-permission android:name="android.permission.CHANGE_WIFI_STATE"></uses-permission> <uses-permission android:name="android.permission.READ_PHONE_STATE"></uses-permission>
- 接下來我們就可以開始編寫百度地图應用程序...待續.
使用 HorizontalScrollView 和 ScrollView 顯示大圖
我們可以使用 HorizontalScrollView 和 ScrollView 顯示比屏幕大的圖像.
<HorizontalScrollView android:layout_width="wrap_content" android:layout_height="wrap_content"> <ScrollView android:layout_width="wrap_content" android:layout_height="wrap_content"> <ImageView android:src="@drawable/bigpicture" android:layout_height="wrap_content" android:layout_width="wrap_content" android:scaleType="center"/> </ScrollView> </HorizontalScrollView>
2012年5月18日星期五
蜂巢(Honeycomb)的對話片段(DialogFragment)
來到 Android 3, 蜂巢(Honeycomb), 為支持應用程序中的多個活動間(multiple activities)的UI和邏輯重用, showDialog()和dismissDialog()方法被棄用, 取而代之的是對話片段(DialogFragment).
實現話片段(DialogFragment), 先創建它的佈局文件和擴展 DialogFragment 類.
實現話片段(DialogFragment)的佈局文件, /res/layout/dialogfragments.xml:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="I'm Dialog Fragment" /> <ImageView android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout>
創建擴展 DialogFragment 的類, MyDialogFragment.java.
package com.Android3DialogFragments; import android.app.DialogFragment; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class MyDialogFragment extends DialogFragment { public MyDialogFragment(){} @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View myDialogFragmentView = inflater.inflate(R.layout.dialogfragments, container); getDialog().setTitle("MyDialogFragment"); return myDialogFragmentView; } }
修改主佈局main.xml, 添加一個按鈕打開對話片段.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> <Button android:id="@+id/opendialogfragment" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Open Dialog Fragment" /> </LinearLayout>
主活動的Java代碼
package com.Android3DialogFragments; import android.app.Activity; import android.app.FragmentManager; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; public class Android3DialogFragmentsActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); Button openDialogFragment = (Button)findViewById(R.id.opendialogfragment); openDialogFragment.setOnClickListener(new OnClickListener(){ @Override public void onClick(View arg0) { openMyDialogFragment(); }}); } private void openMyDialogFragment(){ FragmentManager fragmentManager = getFragmentManager(); MyDialogFragment myDialogFragment = new MyDialogFragment(); myDialogFragment.show(fragmentManager, "tag_myDialogFragment"); } }
2012年5月13日星期日
Android 4, Ice Cream Sandwich (ICS), 的網格佈局(GridLayout)
Android 4, Ice Cream Sandwich (ICS) API Level 14, 提供新的的網格佈局(GridLayout). 注意, 網格佈局(GridLayout)不同於網格視圖(GridView). 下面是一個網格佈局的簡單例子.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> <GridLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:useDefaultMargins="true" android:columnCount="4" android:rowCount="3" > <TextView android:background="@android:color/background_light" android:text="A"/> <TextView android:layout_column="1" android:layout_row="0" android:background="@android:color/background_light" android:text="B"/> <ImageView android:layout_column="2" android:layout_row="0" android:background="@android:color/background_light" android:src="@drawable/ic_launcher"/> <TextView android:layout_column="3" android:layout_row="0" android:background="@android:color/background_light" android:text="D"/> <TextView android:layout_column="1" android:layout_row="1" android:text="F"/> <TextView android:text="G"/> <TextView android:text="H"/> <Button android:layout_columnSpan="3" android:text="I, J, K"/> <TextView android:text="L"/> </GridLayout> </LinearLayout>
Android Developers Blog上有一編講解網格佈局很好的文章.
2012年4月19日星期四
Android 3.0 的彈出菜單(PopupMenu)
Android 3.0, API Level 11, 的彈出菜單(PopupMenu)在一個模態的彈出窗口(modal popup window)之上顯示菜單.
實例:

創建/res/menu/popupmenu.xml文件, 定義彈出菜單(PopupMenu)的佈局.
修改 main.xml 添加一個按鈕啟動彈出菜單.
主Java代碼.
實例:

創建/res/menu/popupmenu.xml文件, 定義彈出菜單(PopupMenu)的佈局.
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<group android:id="@+id/popupmenu">
<item android:id="@+id/menu1"
android:title="item 1"/>
<item android:id="@+id/menu2"
android:title="item 2"/>
<item android:id="@+id/menu3"
android:title="item 3"/>
</group>
</menu>
修改 main.xml 添加一個按鈕啟動彈出菜單.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<Button
android:id="@+id/startpopupmenu"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Start PopupMenu"
/>
</LinearLayout>
主Java代碼.
package com.Android3PopupMenu;
import android.app.Activity;
import android.os.Bundle;
import android.view.MenuItem;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.PopupMenu;
public class Android3PopupMenuActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button buttonPopupMenu = (Button)findViewById(R.id.startpopupmenu);
buttonPopupMenu.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View view) {
// TODO Auto-generated method stub
PopupMenu popupMenu = new PopupMenu(Android3PopupMenuActivity.this, view);
popupMenu.getMenuInflater().inflate(R.menu.popupmenu, popupMenu.getMenu());
popupMenu.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
// Do something...
return true;
}
});
popupMenu.show();
}});
}
}
2012年4月11日星期三
彈出視窗(PopupWindow)
彈出視窗(PopupWindow)就像是一個浮動在主屏幕之上的視窗.
實例:

修改main.xml, 添加一個按鈕用來打開彈出視窗(PopupWindow).
主要的Java代碼.
創建/res/layout/popup.xml文件, 定義彈出視窗的佈局.
實例:

修改main.xml, 添加一個按鈕用來打開彈出視窗(PopupWindow).
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<Button
android:id="@+id/openpopupwindow"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Open PopupWindow" />
</LinearLayout>
主要的Java代碼.
package com.PopupWindow;
import android.app.Activity;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup.LayoutParams;
import android.widget.Button;
import android.widget.PopupWindow;
public class PopupWindowActivity extends Activity {
Button buttonOpen;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
buttonOpen = (Button)findViewById(R.id.openpopupwindow);
buttonOpen.setOnClickListener(buttonOpenOnClickListener);
}
Button.OnClickListener buttonOpenOnClickListener
= new Button.OnClickListener(){
@Override
public void onClick(View view) {
openPopupWindow(view);
}};
private void openPopupWindow(View v){
LayoutInflater layoutInflater = (LayoutInflater)getBaseContext()
.getSystemService(LAYOUT_INFLATER_SERVICE);
View popupView = layoutInflater.inflate(R.layout.popup, null);
final PopupWindow popupWindow = new PopupWindow(popupView,
LayoutParams.WRAP_CONTENT,
LayoutParams.WRAP_CONTENT);
Button buttonClose = (Button)popupView.findViewById(R.id.closepopupwindow);
buttonClose.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
popupWindow.dismiss();
}});
popupWindow.showAsDropDown(buttonOpen);
popupWindow.setFocusable(true);
popupWindow.update();
}
}
創建/res/layout/popup.xml文件, 定義彈出視窗的佈局.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="#000055">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="PopupWindow" />
<Button
android:id="@+id/closepopupwindow"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="OK" />
</LinearLayout>
2012年4月7日星期六
使用managedQuery()獲取Android設備的視頻媒體內容
本程序演示如何使用managedQuery()方法, 從媒體存儲(MediaStore)獲取Android設備的視頻媒體內容. 把Uri設定為 MediaStore.Video.Media.EXTERNAL_CONTENT_URI, 排序設定為 MediaStore.Video.Media.TITLE.


package com.AndroidMediaList;
import android.app.ListActivity;
import android.database.Cursor;
import android.net.Uri;
import android.os.Bundle;
import android.provider.MediaStore;
import android.widget.ListAdapter;
import android.widget.SimpleCursorAdapter;
public class AndroidMediaListActivity extends ListActivity {
//For Video
Uri targetUri = MediaStore.Video.Media.EXTERNAL_CONTENT_URI;
String sortOrder = MediaStore.Video.Media.TITLE;
//For Audio
//Uri targetUri = MediaStore.Audio.Media.EXTERNAL_CONTENT_URI;
//String sortOrder = MediaStore.Audio.Media.TITLE;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
String[] from = {MediaStore.MediaColumns.TITLE};
int[] to = {android.R.id.text1};
Cursor cursor = managedQuery(targetUri, null, null, null, sortOrder);
ListAdapter adapter = new SimpleCursorAdapter(this, android.R.layout.simple_list_item_1, cursor, from, to);
setListAdapter(adapter);
}
}
2012年4月5日星期四
獲取Android設備的音樂媒體內容
本程序演示如何使用managedQuery()方法從媒體存儲(MediaStore)獲取Android設備的音樂媒體內容. 把Uri設定為 MediaStore.Audio.Media.EXTERNAL_CONTENT_URI, 排序設定為 MediaStore.Audio.Media.TITLE.
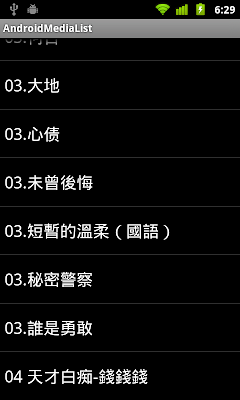
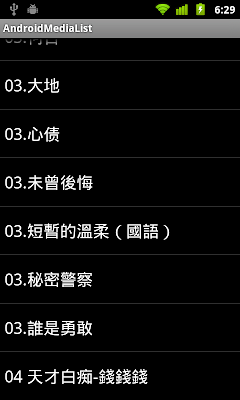
package com.AndroidMediaList;
import android.app.ListActivity;
import android.database.Cursor;
import android.net.Uri;
import android.os.Bundle;
import android.provider.MediaStore;
import android.widget.ListAdapter;
import android.widget.SimpleCursorAdapter;
public class AndroidMediaListActivity extends ListActivity {
Uri targetUri = MediaStore.Audio.Media.EXTERNAL_CONTENT_URI;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
String[] from = {MediaStore.MediaColumns.TITLE};
int[] to = {android.R.id.text1};
Cursor cursor = managedQuery(targetUri, null, null, null, MediaStore.Audio.Media.TITLE);
ListAdapter adapter = new SimpleCursorAdapter(this, android.R.layout.simple_list_item_1, cursor, from, to);
setListAdapter(adapter);
}
}
2012年3月15日星期四
實現透明活動(Transparent Activity)
要令應用程序的背景設定為實現, 可以修改AndroidManifest.xml, 添加
android:theme="@android:style/Theme.Translucent"

android:theme="@android:style/Theme.Translucent"

<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.AndroidProgressDialog"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk android:minSdkVersion="8" />
<application
android:icon="@drawable/ic_launcher"
android:label="@string/app_name" >
<activity
android:name=".AndroidProgressDialog"
android:label="@string/app_name"
android:theme="@android:style/Theme.Translucent">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
2012年3月14日星期三
實現透明進度對話框(Transparent Progress Dialog)
通過自定義對話框, 修改佈局參數的 alpha 屬性, 可以實現透明的進度對話框(Transparent Progress Dialog).

創建 /res/layout/transparentprogressdialog.xml 定義包含進度條(ProgressBar)的對話框.
修改主活動, 實現自定義對話框.
主佈局 main.xml

創建 /res/layout/transparentprogressdialog.xml 定義包含進度條(ProgressBar)的對話框.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical"
android:paddingLeft="100dp"
android:paddingRight="100dp"
android:paddingTop="10dp"
android:paddingBottom="10dp">
<ProgressBar
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
修改主活動, 實現自定義對話框.
package com.AndroidProgressDialog;
import android.app.Activity;
import android.app.Dialog;
import android.os.AsyncTask;
import android.os.Bundle;
import android.os.SystemClock;
import android.view.View;
import android.view.WindowManager;
import android.widget.Button;
public class AndroidProgressDialog extends Activity {
static final int CUSTOM_PROGRESSDIALOG_ID = 0;
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button buttonStart = (Button)findViewById(R.id.start);
buttonStart.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
new asyncTaskUpdateProgress().execute();
}
});
}
@Override
protected Dialog onCreateDialog(int id) {
Dialog dialog = null;
switch(id) {
case CUSTOM_PROGRESSDIALOG_ID:
dialog = new Dialog(AndroidProgressDialog.this);
dialog.setContentView(R.layout.transparentprogressdialog);
dialog.setTitle("ProgressDialog");
WindowManager.LayoutParams params = dialog.getWindow().getAttributes();
params.alpha = 0.5f;
dialog.getWindow().setAttributes(params);
break;
}
return dialog;
}
public class asyncTaskUpdateProgress extends AsyncTask<Void, Integer, Void> {
int progress;
//ProgressDialog progressDialog;
@Override
protected void onPostExecute(Void result) {
// TODO Auto-generated method stub
dismissDialog(CUSTOM_PROGRESSDIALOG_ID);
}
@Override
protected void onPreExecute() {
// TODO Auto-generated method stub
progress = 0;
showDialog(CUSTOM_PROGRESSDIALOG_ID);
}
@Override
protected Void doInBackground(Void... arg0) {
// TODO Auto-generated method stub
while(progress<100){
progress++;
SystemClock.sleep(20);
}
return null;
}
}
}
主佈局 main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/hello" />
<Button
android:id="@+id/start"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="- Start -" />
<ImageView
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:src="@drawable/ic_launcher"/>
</LinearLayout>
2012年3月13日星期二
實現透明的對話框
我們可以通過修改對話框佈局參數的 alpha 屬性, 實現透明的對話框.
WindowManager.LayoutParams params = dialog.getWindow().getAttributes();
params.alpha = 0.6f;
dialog.getWindow().setAttributes(params);

WindowManager.LayoutParams params = dialog.getWindow().getAttributes();
params.alpha = 0.6f;
dialog.getWindow().setAttributes(params);

package com.TransparentDialog;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.view.View;
import android.view.WindowManager;
import android.widget.Button;
public class TransparentDialogActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Button buttonStartDialog = (Button)findViewById(R.id.start);
buttonStartDialog.setOnClickListener(new Button.OnClickListener(){
@Override
public void onClick(View arg0) {
//Create AlertDialog
AlertDialog.Builder myAlertDialog = new AlertDialog.Builder(TransparentDialogActivity.this);
myAlertDialog.setTitle("--- Title ---");
myAlertDialog.setMessage("Alert Dialog Message");
myAlertDialog.setPositiveButton("OK", new DialogInterface.OnClickListener() {
// do something when the button is clicked
public void onClick(DialogInterface arg0, int arg1) {
//...
}});
myAlertDialog.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
// do something when the button is clicked
public void onClick(DialogInterface arg0, int arg1) {
//...
}});
AlertDialog dialog = myAlertDialog.show();
WindowManager.LayoutParams params = dialog.getWindow().getAttributes();
params.alpha = 0.6f;
dialog.getWindow().setAttributes(params);
}});
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button
android:id="@+id/start"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="- Start -" />
<ImageView
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:src="@drawable/ic_launcher"/>
</LinearLayout>
2012年3月10日星期六
自定義佈局的列表片段(ListFragment)
本例子示範如何在列表片段(ListFragment)中顯示自定義的佈局.

創建/res/layout/row.xml文件, 定義每一個列的佈局.
修改MyListFragment.java, 創建和使用我們自定義的陣列適配器, MyCustomAdapter.
其他文件, Android3ListFragmentActivity.java(主Activity), main.xml(主layout), listfragment.xml, 參考前文"Android 3.0 的列表片段(ListFragment)".

創建/res/layout/row.xml文件, 定義每一個列的佈局.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/text1"
android:textSize="26dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/text2"
android:textSize="26dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
修改MyListFragment.java, 創建和使用我們自定義的陣列適配器, MyCustomAdapter.
package com.Android3ListFragment;
import android.app.ListFragment;
import android.content.Context;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.TextView;
public class MyListFragment extends ListFragment {
class Month{
String chinese;
String english;
Month(String c, String e){
chinese = c;
english = e;
}
public String getChinese(){
return chinese;
}
public String getEnglish(){
return english;
}
}
Month[] months ={
new Month("一月", "January"),
new Month("二月", "February"),
new Month("三月", "March"),
new Month("四月", "April"),
new Month("五月", "May"),
new Month("六月", "June"),
new Month("七月", "July"),
new Month("八月", "August"),
new Month("九月", "September"),
new Month("十月", "October"),
new Month("十一月", "November"),
new Month("十二月", "December")};
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.listfragment, container, false);
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setListAdapter(new MyCustomAdapter(
getActivity(),
R.layout.row,
months));
}
public class MyCustomAdapter extends ArrayAdapter<Month>{
public MyCustomAdapter(Context context, int textViewResourceId,
Month[] objects) {
super(context, textViewResourceId, objects);
// TODO Auto-generated constructor stub
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
// TODO Auto-generated method stub
LayoutInflater inflater=getActivity().getLayoutInflater();
View row=inflater.inflate(R.layout.row, parent, false);
TextView text1 = (TextView)row.findViewById(R.id.text1);
TextView text2 = (TextView)row.findViewById(R.id.text2);
text1.setText(months[position].getChinese());
text2.setText(months[position].getEnglish());
return row;
}
}
}
其他文件, Android3ListFragmentActivity.java(主Activity), main.xml(主layout), listfragment.xml, 參考前文"Android 3.0 的列表片段(ListFragment)".
2012年3月8日星期四
AIDE - Android Java IDE: 在Android上運行的Android開發工具

AIDE - Android Java IDE Android App是一個直接在Android設備上開發Android Apps的集成開發環境(IDE). 它支持完整的編輯 - 編譯 - 運行週期: 功能豐富的編輯器, 提供先進的功能, 如代碼完成(code completion), 實時錯誤檢查(real-time error checking), 重構和智能代碼導航和運行應用程序.
AIDE完全兼容的Eclipse項目.
Google play 連結>>>
AIDE在Galaxy S2上運行的示範視頻:
MIT App Inventor測試版

早前 Google 開發的 App Inventor 轉移到麻省理工(Massachusetts Institute of Technology, MIT)而停了一段時間. 現在正名為 MIT App Inventor, 是麻省理工學院移動學習中心(MIT Center for Mobile Learning)的一個項目. 剛剛發佈測試版.
連結: http://appinventor.mit.edu/

2012年3月6日星期二
Android 3.0 的列表片段(ListFragment)

- 建立新的XML文件, /res/layout/listfragment.xml.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#101010">
<ListView android:id="@id/android:list"
android:layout_width="match_parent"
android:layout_height="0dip"
android:layout_weight="1"/>
<TextView android:id="@id/android:empty"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="No data"/>
</LinearLayout>
- 擴展ListFragment, 建立新的MyListFragment.java類.
package com.Android3ListFragment;
import android.app.ListFragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
public class MyListFragment extends ListFragment {
String[] month ={
"January", "February", "March", "April",
"May", "June", "July", "August",
"September", "October", "November", "December"};
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.listfragment, container, false);
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setListAdapter(new ArrayAdapter<String>(
getActivity(),
android.R.layout.simple_list_item_1,
month));
}
}
- 修改main.xml.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="horizontal" >
<TextView
android:layout_height="wrap_content"
android:layout_width="0px"
android:layout_weight="3"
android:text="@string/hello" />
<fragment
class="com.Android3ListFragment.MyListFragment"
android:id="@+id/mylistfragment"
android:layout_width="0px"
android:layout_weight="1"
android:layout_height="match_parent"/>
</LinearLayout>
主Activity使用自動生成的代碼便可以:
package com.Android3ListFragment;
import android.app.Activity;
import android.os.Bundle;
public class Android3ListFragmentActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
}
相關文章:
- 自定義佈局的列表片段(ListFragment)
2012年2月29日星期三
2012年2月25日星期六
2012年2月24日星期五
新的標誌, 新的 developers.google.com

谷歌正在努力透過集合所有開發資源, 計劃, 活動, 工具, 和社區到一個地方 developers.google.com, 給開發者社區提供更好的支持.
新的標誌, 一個新的身份, 以完整嶄新的面貌, 統一 Google 的開發產品. 它反映了關注的焦點是在開發者, 不只是開發的工具.
know more: developers.google.com - Google Developers
2012年2月9日星期四
使用 Java 程序碼實現動作欄(Action Bar)
前面一些文章示範如何通過 XML 文件定義動作欄(Action Bar). 這篇文章示範如何使用 Java 程序碼實現動作欄(Action Bar).
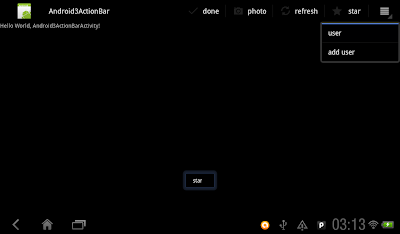
像下面修改 Activity 中的 onCreateOptionsMenu(Menu menu) 方法實現動作欄, 同時修改 onOptionsItemSelected(MenuItem item) 的 switch-case 處理對應的數值.
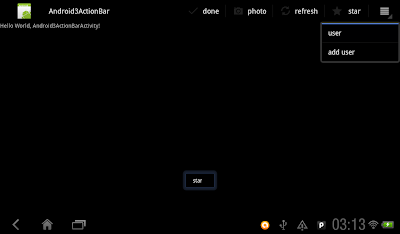
像下面修改 Activity 中的 onCreateOptionsMenu(Menu menu) 方法實現動作欄, 同時修改 onOptionsItemSelected(MenuItem item) 的 switch-case 處理對應的數值.
package com.Android3ActionBar;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.Toast;
public class Android3ActionBarActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
super.onCreateOptionsMenu(menu);
MenuItem menuItem0 = menu.add(0, 0, 0, "done");
//(groupId, itemId, order, title)
menuItem0.setIcon(R.drawable.ic_action_done);
menuItem0.setShowAsAction(MenuItem.SHOW_AS_ACTION_IF_ROOM
|MenuItem.SHOW_AS_ACTION_WITH_TEXT);
MenuItem menuItem1 = menu.add(0, 1, 1, "photo");
menuItem1.setIcon(R.drawable.ic_action_photo);
menuItem1.setShowAsAction(MenuItem.SHOW_AS_ACTION_IF_ROOM
|MenuItem.SHOW_AS_ACTION_WITH_TEXT);
MenuItem menuItem2 = menu.add(0, 2, 2, "refresh");
menuItem2.setIcon(R.drawable.ic_action_refresh);
menuItem2.setShowAsAction(MenuItem.SHOW_AS_ACTION_IF_ROOM
|MenuItem.SHOW_AS_ACTION_WITH_TEXT);
MenuItem menuItem3 = menu.add(0, 3, 3, "star");
menuItem3.setIcon(R.drawable.ic_action_star);
menuItem3.setShowAsAction(MenuItem.SHOW_AS_ACTION_IF_ROOM
|MenuItem.SHOW_AS_ACTION_WITH_TEXT);
MenuItem menuItem4 = menu.add(0, 4, 4, "user");
menuItem4.setIcon(R.drawable.ic_action_user);
menuItem4.setShowAsAction(MenuItem.SHOW_AS_ACTION_IF_ROOM
|MenuItem.SHOW_AS_ACTION_WITH_TEXT);
MenuItem menuItem5 = menu.add(0, 5, 5, "add user");
menuItem5.setIcon(R.drawable.ic_action_user_add);
menuItem5.setShowAsAction(MenuItem.SHOW_AS_ACTION_IF_ROOM
|MenuItem.SHOW_AS_ACTION_WITH_TEXT);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// TODO Auto-generated method stub
switch(item.getItemId()){
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
Toast.makeText(Android3ActionBarActivity.this,
item.getTitle(),
Toast.LENGTH_LONG).show();
return true;
default:
return false;
}
}
}
2012年2月3日星期五
處理動作欄(Action Bar)的用戶選項操作, onOptionsItemSelected()
重寫onOptionsItemSelected(MenuItem item)方法, 我們可以處理用戶的動作欄(Action Bar)選項.


package com.Android3ActionBar;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.Toast;
public class Android3ActionBarActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu, menu);
return super.onCreateOptionsMenu(menu);
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// TODO Auto-generated method stub
switch(item.getItemId()){
case R.id.itemid_0:
case R.id.itemid_1:
case R.id.itemid_2:
case R.id.itemid_3:
case R.id.itemid_4:
case R.id.itemid_5:
Toast.makeText(Android3ActionBarActivity.this,
item.getTitle(),
Toast.LENGTH_LONG).show();
return true;
default:
return false;
}
}
}
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/itemid_0"
android:title="Done"
android:orderInCategory="0"
android:icon="@drawable/ic_action_done"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_1"
android:title="Photo"
android:orderInCategory="0"
android:icon="@drawable/ic_action_photo"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_2"
android:title="Refresh"
android:orderInCategory="0"
android:icon="@drawable/ic_action_refresh"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_3"
android:title="Star"
android:orderInCategory="0"
android:icon="@drawable/ic_action_star"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_4"
android:title="User"
android:orderInCategory="0"
android:icon="@drawable/ic_action_user"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_5"
android:title="Add User"
android:orderInCategory="0"
android:icon="@drawable/ic_action_user_add"
android:showAsAction="ifRoom|withText" />
</menu>
2012年2月2日星期四
添加動作欄(Action Bar)圖標(icon)


修改/res/menu/menu.xml文件, 在 <item> 加入 android:icon 和 android:showAsAction 屬性.
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/itemid_0"
android:title="Done"
android:orderInCategory="0"
android:icon="@drawable/ic_action_done"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_1"
android:title="Photo"
android:orderInCategory="0"
android:icon="@drawable/ic_action_photo"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_2"
android:title="Refresh"
android:orderInCategory="0"
android:icon="@drawable/ic_action_refresh"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_3"
android:title="Star"
android:orderInCategory="0"
android:icon="@drawable/ic_action_star"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_4"
android:title="User"
android:orderInCategory="0"
android:icon="@drawable/ic_action_user"
android:showAsAction="ifRoom|withText" />
<item android:id="@+id/itemid_5"
android:title="Add User"
android:orderInCategory="0"
android:icon="@drawable/ic_action_user_add"
android:showAsAction="ifRoom|withText" />
</menu>
相關文章:
- 通過 XML 創建 Android 3.0 的操作欄(ActionBar)
- 下載 Android 為操作欄(action bar)提供的圖標(action icons)
- 處理動作欄(Action Bar)的用戶選項操作, onOptionsItemSelected()
2012年1月31日星期二
下載 Android 為操作欄(action bar)提供的圖標(action icons)
為了進一步在操作欄(action bar)中提供一個一致的用戶體驗, Android UX 團隊提供一些預先設計的動作圖標(action icons), 以供使用. 這些圖標專為 light 以及 dark Holo 主題而設, 支持常見的用戶操作, 如刷新(Refresh), 刪除(Delete), 附加(Attach), 星(Star), 分享(Share)...等等, 可以從這裡下載.


Android 開發人員的 Google+ 專頁

Google 宣布推出 Android 開發人員的 Google+ 專頁 - +Android Developers.
+Android Developers 專頁為世界各地的開發者提供一個聚會和討論最新應用程序開發信息的地方. 谷歌會在+Android Developers 專頁發表有關開發技巧,SDK和開發工具,Android培訓班的信息, 以及來自世界各地 Android 開發者活動的視頻和圖片.
了解更多: Android Developers Blog - Android Developers on Google+
2012年1月30日星期一
通過 XML 創建 Android 3.0 的操作欄(ActionBar)

先創建 /res/menu/menu.xml 定義操作欄(ActionBar).
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/itemid_0"
android:title="Action Item 0"
android:orderInCategory="0" />
<item android:id="@+id/itemid_1"
android:title="Action Item 1"
android:orderInCategory="0" />
<item android:id="@+id/itemid_2"
android:title="Action Item 2"
android:orderInCategory="0" />
<item android:id="@+id/itemid_3"
android:title="Action Item 3"
android:orderInCategory="0" />
</menu>
在Java主代碼中重寫 onCreateOptionsMenu(Menu menu) 方法, 使用 getMenuInflater().inflate(R.menu.menu, menu) 實現操作欄(ActionBar).
package com.Android3ActionBar;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
public class Android3ActionBarActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu, menu);
return super.onCreateOptionsMenu(menu);
}
}
相關文章:
- 添加動作欄(Action Bar)圖標(icon)
2012年1月24日星期二
通過XML文件, 創建片段(Fragment)
前文"Android 3.0 新增的片段(Fragment)"的MyFragment.java類使用Java程序碼創建片段(Fragment). 本例修改MyFragment.java, 使用inflater類的inflate()方法, 通過XML文件, 創建片段(Fragment).

- 建立新的XML文件, /res/layout/fragment1.xml
- 修改MyFragment.java
- 沿用前文的main.xml

- 建立新的XML文件, /res/layout/fragment1.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="FRAGMENT HERE" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_launcher"/>
</LinearLayout>
- 修改MyFragment.java
package com.android3Frag;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class MyFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment1, container, false);
return view;
}
}
- 沿用前文的main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="horizontal" >
<TextView
android:layout_width="0px"
android:layout_weight="2"
android:layout_height="match_parent"
android:text="@string/hello"/>
<fragment
class="com.android3Frag.MyFragment"
android:id="@+id/myfragment"
android:layout_width="0px"
android:layout_weight="3"
android:layout_height="match_parent"/>
</LinearLayout>
2012年1月16日星期一
Android 3.0 新增的片段(Fragment)
Android 3.0 新增片段(Fragment), 可以理解為類似嵌入活動(activity)內的一個子活動.
這是一個簡單的例子:

在Eclipse創建一個新項目, Android3Frag; 要注意是: 建設目標(Build Targe)必須是 Android 3.0 (API Level 11)或更高.
先通過擴展 Fragment 創建一個 MyFragment 類, 並且重寫onCreateView()方法. 這便是我們的片段(Fragment).
修改佈局, main.xml.
相關文章:
- 通過XML文件, 創建片段(Fragment)
這是一個簡單的例子:

在Eclipse創建一個新項目, Android3Frag; 要注意是: 建設目標(Build Targe)必須是 Android 3.0 (API Level 11)或更高.
先通過擴展 Fragment 創建一個 MyFragment 類, 並且重寫onCreateView()方法. 這便是我們的片段(Fragment).
package com.android3Frag;
import android.app.Fragment;
import android.content.Context;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
public class MyFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
Context context = getActivity().getApplicationContext();
LinearLayout layout = new LinearLayout(context);
TextView text = new TextView(context);
text.setText("FRAGMENT HERE");
ImageView image = new ImageView(context);
image.setImageDrawable(getResources().getDrawable(R.drawable.ic_launcher));
layout.addView(text);
layout.addView(image);
return layout;
}
}
修改佈局, main.xml.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="horizontal" >
<TextView
android:layout_width="0px"
android:layout_weight="2"
android:layout_height="match_parent"
android:text="@string/hello"/>
<fragment
class="com.android3Frag.MyFragment"
android:id="@+id/myfragment"
android:layout_width="0px"
android:layout_weight="3"
android:layout_height="match_parent"/>
</LinearLayout>
相關文章:
- 通過XML文件, 創建片段(Fragment)
2012年1月13日星期五
谷歌發布Android設計網站 (Android Design)

谷歌推出Android設計網站(Android Design), 幫助學習創建Android用戶界面的設計原則, 構建組件塊, 以及設計模式. 無論你是UI專業人才或是開發用戶界面的開發人員,這些文件告訴你如何使良好的設計決策。
詳細參考: Android Developers Blog - Introducing the Android Design site
2012年1月11日星期三
訂閱:
文章 (Atom)